Configuration description
Environment variables
Variables | Parameters | Description |
---|---|---|
BOT_TOKEN | string | Your discord bot token |
Configure the config.js file
/**
* @type {import('./src/@types/index.js').Config} - Bot config
*/
const config = {
// Lavalink node list
nodeList: [
{
id: 'Node 1',
hostname: 'localhost',
port: 2333,
password: 'youshallnotpass'
}
],
spotify: {
clientId: '', // If you want to use Spotify to play songs, you need to set up Spotify credentials.
clientSecret: '' // https://developer.spotify.com/documentation/web-api
},
bot: {
textCommand : true, // Whether to enable text command
slashCommand : true, // Whether to enable slash command
// OAUTH2 mode requires setting 'admin', 'clientSecret' value
admin : [], // Admin users, It must be the user ID (string[])
dj : [], // DJ users, It must be the user ID (string[])
djRoleId : '', // DJ role ID, members with this role have DJ permissions (string)
clientSecret : '',
name : 'Music Disc',
prefix : '+',
status : 'online', // 'online' | 'idle' | 'dnd'
activity: {
type : 0, // https://discord.com/developers/docs/topics/gateway-events#activity-object-activity-types
name : '+help | music',
// state : '',
// url : '', // The streaming type currently only supports Twitch and YouTube. Only https://twitch.tv/ and https://youtube.com/ urls will work.
},
embedsColor : '#FFFFFF',
volume: {
default : 50,
max : 100,
},
// Auto leave channel settings
autoLeave: {
enabled : true,
cooldown : 5000, // ms
},
// Show voice channel updates
displayVoiceState : true,
// Specify the text channel for receiving commands.
// If this value is set, text messages from other channels will not be processed.
specifyMessageChannel : '', // Text channel ID
// Specify the voice channel to join.
// If this value is set, other voice channels will not be joined.
specifyVoiceChannel : '', // Vioce channel ID
// After starting the Bot, it will automatically join the specified voice channel and wait.
// The specifyVoiceChannel value needs to be set, otherwise it will be invalid.
startupAutoJoin : false,
// Language settings
i18n: {
localePath : '../../locales',
defaultLocale : 'en-US'
}
},
blacklist : [], // It must be the user ID (string[])
// Web dashboard settings
webDashboard: {
enabled : true,
port : 33333,
loginType : 'USER', // 'USER' | 'OAUTH2'
// USER mode settings
user: {
username : 'admin',
password : 'password',
},
// OAUTH2 mode settings
oauth2: {
link : '',
redirectUri : 'http://localhost:33333/login',
},
// SessionManager config
sessionManager: {
validTime : 10 * 60 * 1000, // Session validity time (ms) (default: 10 minutes)
cleanupInterval : 5 * 60 * 1000 // Timing cleaner time (ms) (default: 5 minutes)
},
// IPBlocker config
ipBlocker: {
retryLimit : 5, // Maximum number of retries (default: 5)
unlockTimeoutDuration : 5 * 60 * 1000, // Blocking time (ms) (default: 5 minutes)
cleanupInterval : 5 * 60 * 1000 // Timing cleaner time (ms) (default: 5 minutes)
}
},
// Local Lavalink node
localNode: {
enabled : false,
autoRestart : true,
// downloadLink : 'https://github.com/lavalink-devs/Lavalink/releases/download/4.0.8/Lavalink.jar'
},
// Command permission settings
command: {
disableCommand: [], // Disabled commands, all enabled by default
adminCommand: ['language','server', 'status'], // Admin commands, only Admin role user can use
djCommand: [] // DJ commands, only DJ role user can use
}
};
export { config };
Lavalink Node List
Detailed node parameter documentation: https://lavashark.js.org/docs/types/Node.types#nodeoptions
Property | Type | Description |
---|---|---|
id | string | Identifier for the node (must be unique) |
hostname | string | Hostname or IP of the Lavalink node |
port | number | Port on which the node is listening |
password | string | Password for authenticating with the node |
Spotify Credentials
If Spotify Credentials is not set, anonymous token is used by default.
Spotify Development Documentation: https://developer.spotify.com/documentation/web-api
Property | Type | Description |
---|---|---|
clientId | string | Spotify API client ID |
clientSecret | string | Spotify API client secret |
Bot Settings
Property | Type | Description |
---|---|---|
textCommand | boolean | Enable or disable legacy text-based commands (+play , etc.) |
slashCommand | boolean | Enable or disable Discord slash commands (/play , etc.) |
admin | string[] | User IDs with full admin permissions |
dj | string[] | User IDs with DJ-level permissions |
djRoleId | string | Role ID that grants DJ permissions to its members |
clientSecret | string | OAuth2 client secret for Discord (required if using OAUTH2 login web dashboard) |
name | string | Bot display name |
prefix | string | Prefix for text commands (e.g. +play , +skip ) |
status | online , idle , dnd | Bot presence status (online , idle , or dnd ) |
-- | -- | -- |
activity | ||
activity.type | number | Discord Activity Type (Discord API docs: https://discord.com/developers/docs/topics/gateway-events#activity-object-activity-types) |
activity.name | string | Bot activity description |
activity.state | string | Optional activity state (Displays under the activity name) |
activity.url | string | Streaming URL for Twitch or YouTube when using streaming activity type (Only https://twitch.tv/ and https://youtube.com/ urls will work.) |
-- | -- | -- |
embedsColor | string | HEX color code for embed messages |
-- | -- | -- |
volume | ||
volume.default | number | Default playback volume (0–100) |
volume.max | number | Maximum allowed volume (0–100) |
-- | -- | -- |
autoLeave | ||
autoLeave.enabled | boolean | Automatically leave voice channel when playback finishes |
autoLeave.cooldown | number | Delay (in milliseconds) before auto-leaving |
-- | -- | -- |
displayVoiceState | boolean | Display join/leave voice state updates in chat |
specifyMessageChannel | string | Text channel ID to restrict command input (leave empty to allow all channels) |
specifyVoiceChannel | string | Voice channel ID to restrict bot joins (leave empty to allow any channel) |
startupAutoJoin | boolean | Automatically join the specified voice channel on startup |
-- | -- | -- |
i18n | ||
i18n.localePath | string | Relative path to localization files |
i18n.defaultLocale | string | Default locale for messages (e.g. en-US ) |
Blacklist
You can add the user IDs you want to block to the blacklist.json array.
Commands issued by users added to the blacklist will be ignored,
and when such a user joins a voice channel, the bot will automatically leave or will not enter the channel.
Property | Type | Description |
---|---|---|
blacklist | string[] | User IDs that are blocked; commands and auto-joins are ignored for these IDs |
examples:
blacklist: ['696000000297000372', '1310000644500000084']
Web Dashboard
Property | Type | Description |
---|---|---|
enabled | boolean | Enable or disable the web dashboard |
port | number | Port on which the dashboard listens |
loginType | 'USER' | Authentication mode (USER or OAUTH2 ) |
-- | -- | -- |
USER mode | ||
user.username | string | Username for dashboard login (USER mode) |
user.password | string | Password for dashboard login (USER mode) |
-- | -- | -- |
OAUTH2 mode | OAUTH2 mode description | |
oauth2.link | string | OAuth2 authorization URL |
oauth2.redirectUri | string | Redirect URI registered in Discord OAuth2 settings |
-- | -- | -- |
sessionManager | ||
sessionManager.validTime | number | Session validity duration in milliseconds (default: 10 minutes) |
sessionManager.cleanupInterval | number | Interval for cleaning expired sessions in milliseconds (default: 5 minutes) |
-- | -- | -- |
ipBlocker | ||
ipBlocker.retryLimit | number | Maximum consecutive failed login attempts before blocking in milliseconds |
ipBlocker.unlockTimeoutDuration | number | Duration the IP remains blocked after reaching retry limit in milliseconds (default: 5 minutes) |
ipBlocker.cleanupInterval | number | Interval for cleaning expired IP blocks in milliseconds (default: 5 minutes) |
Local Lavalink Node
Local node is a lavalink node setting that can be run locally.
There is no need to find a public node or self-hosted.
Just set localNode.enabled
to true to enable it. (requires java17 or newer required)
When enabled, the lavalink node will be downloaded from the default download link
or the manually set localNode.downloadLink
link to run.
This bot only supports Lavalink v4 nodes.
v4.0.8 or higher is recommended.
Property | Type | Description |
---|---|---|
enabled | boolean | Whether to download and run a local Lavalink node |
autoRestart | boolean | Automatically restart the node if it crashes |
downloadLink | string | Optional custom download URL for the Lavalink JAR (default source: https://github.com/lavalink-devs/Lavalink/releases/download/4.0.8/Lavalink.jar) |
Command Permissions
Property | Type | Description |
---|---|---|
disableCommand | string[] | List of commands to disable (all enabled by default) |
adminCommand | string[] | Commands restricted to admin users |
djCommand | string[] | Commands restricted to DJ users |
Oauth2 login
If you want to use oauth2 to log in to the dashboard,
please modify the webDashboard.loginType
value to 'OAUTH2'
and set the bot.admin
, bot.clientSecret
, webDashboard.oauth2.link
, webDashboard.oauth2.redirectUri
values to enable it.
Open the developer portal to the robot's oauth2 settings, obtain the client key and set it to BOT_CLIENT_SECRET
,
and set the redirect url (webDashboard.oauth2.redirectUri
must be exactly the same as this value).
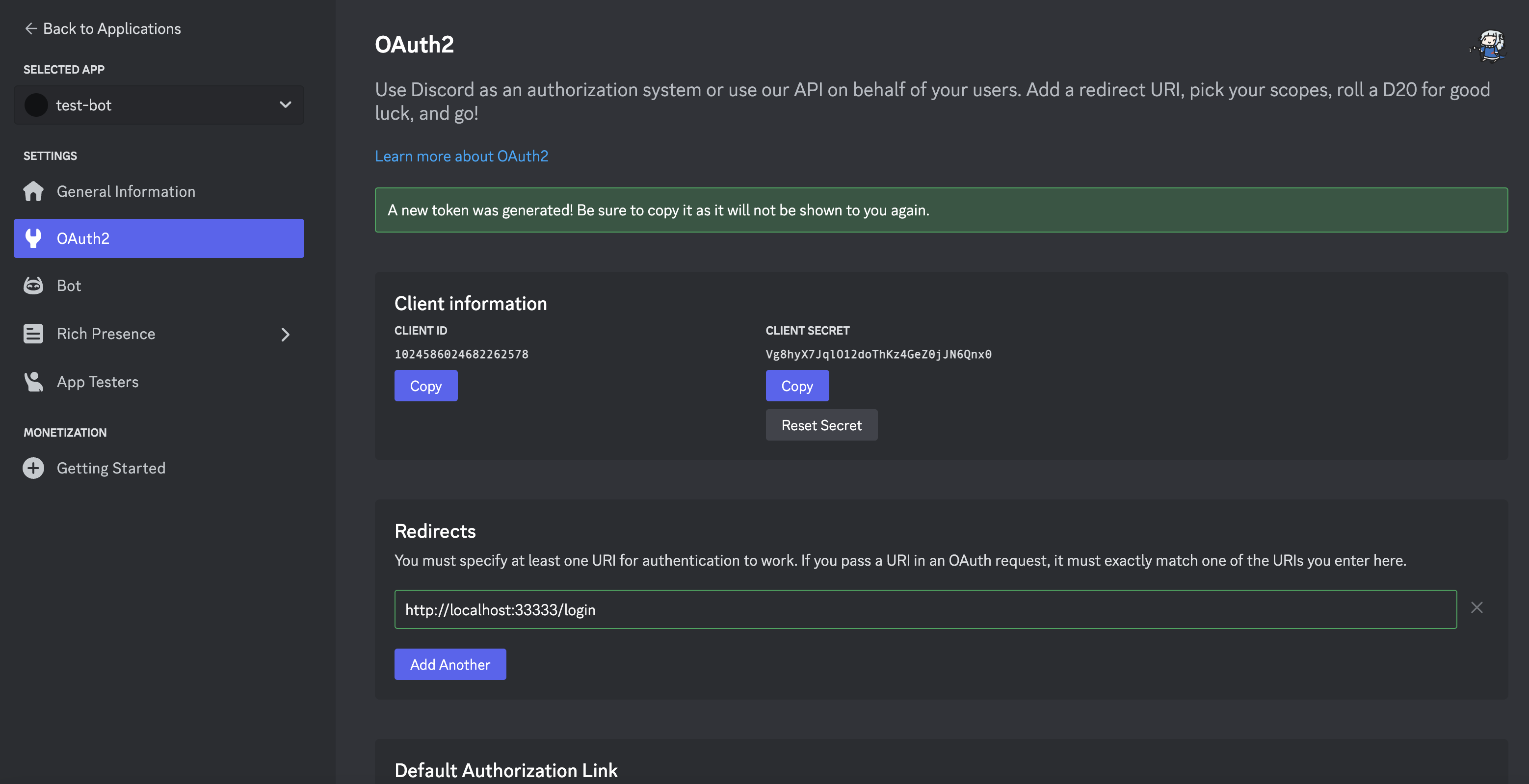
The SITE_OAUTH2_LINK
value is generated using the OAuth2 URL Generator below. For scopes, just select identify.
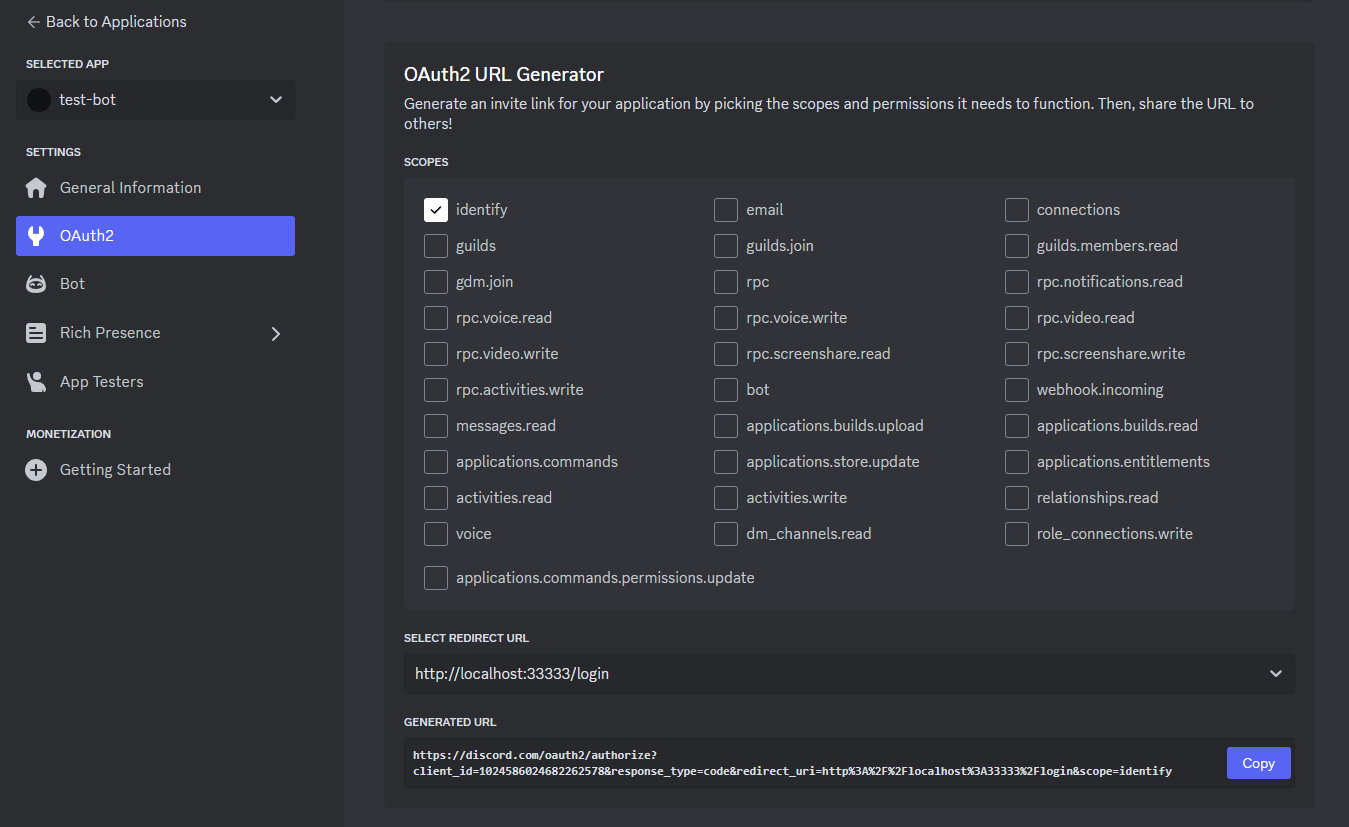